This article explains to DEMONSTRATE HIERARCHICAL INHERITANCE.
DEMONSTRATE HIERARCHICAL INHERITANCE.
Also, you can use the Java compiler to compile a program.
Source code.
import java.util.*;
class employee
{
int age;
String name,addr,gender;
void employee()
{
Scanner s=new Scanner(System.in);
System.out.println("Enter the Name of Employee");
name=s.next();
System.out.println("Enter the gender of Employee");
gender=s.next();
System.out.println("Enter the Addr of Employee");
addr=s.next();
System.out.println("Enter the age of Employee");
age=s.nextInt();
} // END OF METHOD
void disp()
{
System.out.println("Name: "+name);
System.out.println("age: "+age);
System.out.println("Gender: "+gender);
System.out.println("Addr: "+addr);
} // END OF METHOD
}//END OF CLASS
class fulltime extends employee
{ int sal;
String dest;
void full()
{
Scanner s=new Scanner(System.in);
System.out.println("Enter the destination of Employee");
dest=s.next();
System.out.println("Enter the Salary");
sal=s.nextInt();
}
void disp()
{
System.out.println("destination: "+dest);
System.out.println("Salary: "+sal);
}
}//END OF CLASS
class parttime extends employee
{
int work , rate;
void part()
{
Scanner s=new Scanner(System.in);
System.out.println("Enter the working of hours:");
work=s.nextInt();
}
void cal()
{
rate = 8 * work;
}
void disp()
{
System.out.println(" Workthours: " +work);
System.out.println("Rate: " +rate);
}
}//END OF CLASS
class hier
{
public static void main(String args[])
{
int ch,n;
Scanner s=new Scanner(System.in);
do{
System.out.println("1.full time:\n2.part time\n");
System.out.println("Enter the choice:");
ch=s.nextInt();
employee z = new employee(); // CREATING OBJECT
fulltime y = new fulltime(); // CREATING OBJECT
parttime x = new parttime(); // CREATING OBJECT
switch(ch)
{
case 1: System.out.println(" Enter Full Time Employee Details
\n");
System.out.println("==============================\n");
z.employee();
y.full();
System.out.println("Full Time Employee Details \n");
System.out.println("==============================\n");
z.disp();
y.disp();
break;
case 2 :System.out.println(" Enter Part Time Employee Details\n");
System.out.println("==============================\n");
z.employee();
x.part();
x.cal();
System.out.println("Part Time Employee Details\n");
System.out.println("==============================\n");
z.disp();
x.disp();
break;
}//END OF SWITCH
System.out.println("Do you want to
continue\n1.yes\n2.no\n");
n=s.nextInt();
}while(n==1);
} // END OF MAIN
} //END OF CLASS
Output.
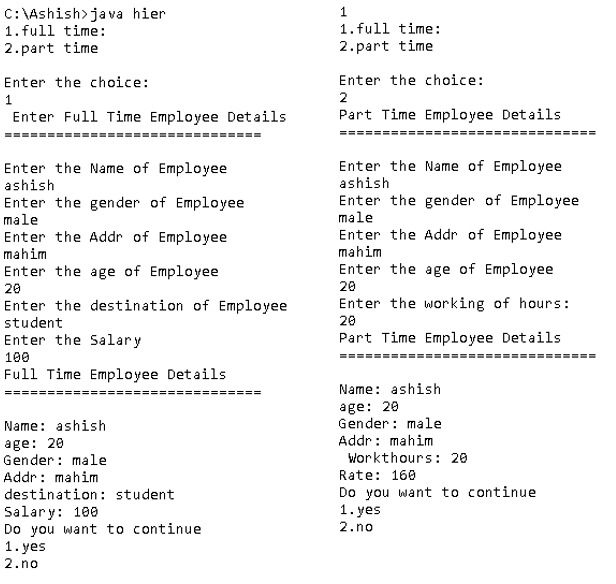
Also View: – Write a program to create a registration form using AWT.
Also View- Write a java program to create a window using swing.