A Deque or deck is a Double Ended Queue. Allows elements to be added or removed on either the ends. Deque is a generalized version of a Queue data structure that allows insert and delete at both ends.
Operation in Double Ended Queue
- Insert an element at the back
- Insert an element at the front
- Remove element at front
- Remove element at the back
Graphical Representation.
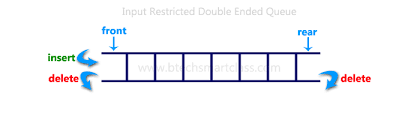
Program for Deque.
view more:-Implementation of Quick Sort| Merge Sort| Heap Sort Algorithm.
Source code.
/* dequeue*/
#include<stdio.h>
#include<stdlib.h>
struct node
{
int data;
struct node *addr;
};
struct node *rear,*head;
void fdel();
void rdel();
void ins(int);
void disp();
int main()
{
ins(10);
ins(20);
ins(30);
ins(40);
printf("Delete element from last\n");
rdel(); //delete element from last
rdel(); //delete elemeny from last
printf("Delete element from last\n");
fdel(); //delete element fron first
fdel();//delete element fron first
fdel();
}
void ins (int val)
{
struct node *temp;
temp=(struct node *)malloc(sizeof(struct node));
temp->data=val;
temp->addr=NULL;
if (head==NULL)
{
head=temp;
rear=temp;
}
else
{
rear->addr=temp;
rear=temp;
}
disp();
}
void disp()
{
struct node *i=head;
printf("queue: ");
while(i!=NULL)
{
printf("%d ",i->data);
i=i->addr;
}
printf("\n ");
}
void fdel()
{
//if queue is empty
if(head==NULL)
printf("Error:empty\n");
//else
else
{
//more than one node exist
head=head->addr;
//only one node exist
if(head==NULL)
rear=NULL;
}
//display
disp();
}
void rdel()
{
struct node *i=head;
if(head!=NULL)
{
while(i->addr!=rear)
{
i=i->addr;
}
i->addr=NULL;
rear=i;
if(rear==NULL)
{
head=NULL;
}
disp();
}
} /* dequeue*/
Output.
queue: 10
queue: 10 20
queue:10 20 30
queue: 10 20 30 40
Delete element from last
queue: 10 20 30
queue: 10 20
Delete element from last
queue: 20
queue:
Error:empty
queue:
Source:-greekforgreek